Godot GDScript Optimization In this article, we’ll share the top 10 GDScript tips for improving performance in your games and apps.
1. Store Data Using Dictionaries and Arrays Instead of Nodes
Storing data in nodes can be inefficient, as it requires the creation of a new object for each piece of data. This can lead to slower performance, especially if you need to store a large amount of data. Instead, consider using dictionaries and arrays to store data. Dictionaries are key-value pairs that allow you to access data using a unique key, while arrays are ordered lists of data that you can access using an index. Both dictionaries and arrays are faster and more efficient than nodes, and they allow you to access and manipulate data without the overhead of object creation. For example, let’s say you need to store a list of high scores for a game. Using nodes, you might create a “HighScore” node for each score and store it in a “HighScores” node. However, this would create a new object for each score, which could be slow if you have a large number of scores. Instead, you could use an array to store the scores and access them using an index. This would be faster and more efficient, as you would only need to create one object for the array, rather than one object for each score.
2. Avoid Using Getters and Setters
Getters and setters are used to access and modify object properties in GDScript. A getter is a function that is called when you access a property, while a setter is a function that is called when you modify a property. While getters and setters can be useful in some cases, they can also be slow, as they require an additional function call every time a property is accessed or modified. This can lead to slower performance, especially if you access or modify properties frequently. To improve performance, consider avoiding getters and setters and accessing properties directly instead. For example, let’s say you have a “Health” property that you want to access and modify in your game. Rather than using a getter and setter, you could simply access the property directly, like this: By accessing properties directly, you can avoid the overhead of function calls and improve performance. (Note: It is generally best practice to use getters and setters for properties that need additional processing)
3. Use Object Pooling
Object pooling is a technique that involves reusing objects rather than constantly creating and destroying them. This can be especially useful in GDScript when you are working with large numbers of objects that are frequently created and destroyed, such as bullets in a game or particles in a particle system. By pooling objects, you can reduce the overhead of constantly creating and destroying objects, which can improve the performance of your script. To implement object pooling in GDScript, you can create a pool of objects that are not being used, and then reuse those objects when you need them instead of creating new ones. When you are finished with an object, you can return it to the pool for reuse later. This can help to reduce the number of objects that need to be created and destroyed, which can improve the performance of your script. Here is an example of how you might use object pooling in GDScript: By using object pooling in this way, you can reduce the overhead of constantly creating and destroying bullet objects and improve the performance of your script.
4. Avoid Using Global Variables
Global variables are variables that are available to all objects in the game. While they can be convenient, they can also be slow, as they require an additional lookup every time they are accessed. This can lead to slower performance, especially if you access global variables frequently. To improve performance, consider avoiding global variables and using local variables instead. Local variables are variables that are defined within a function or block of code and are only available within that scope. They are faster than global variables, as they don’t require an additional lookup when they are accessed. For example: By using local variables, you can avoid the overhead of global variable lookups and improve performance.
5. Use Static Functions
Static functions are functions that are associated with a class, rather than an instance of the class. They can be faster than non-static functions, as they don’t require the overhead of object creation. To use static functions, simply prefix the function with the “static” keyword. For example, let’s say you have a “Player” class that has a “health” property and a “take_damage” function. To make these functions, you could do the following: By using static functions and variables, you can avoid the overhead of object creation and improve performance.
6. Use C# or C++ for Performance-Critical Code
While GDScript is a powerful and easy-to-use language, it may not always be the fastest option for performance-critical code. If you need to optimize specific functions or algorithms, consider using C# or C++ instead. Both languages can be used with the Godot engine, and they offer more control and performance than GDScript. To use C# or C++ with the Godot engine, you will need to install the relevant language support and create a new C# or C++ script. Then, you can define functions and variables in the script and call them from GDScript. Here is an example of how to call a C# function from GDScript: By using C# or C++ for performance-critical code, you can take advantage of the performance and control offered by these languages and optimize specific functions or algorithms in your game.
7. Optimize Loops
Loops are a common source of performance bottlenecks in GDScript code. To optimize loops, consider using the “for” loop instead of the “while” loop, as it is generally faster. You can also improve performance by pre-allocating arrays, using the “continue” and “break” statements wisely, and minimizing the number of iterations. For example, let’s say you have a “while” loop that iterates over a list of items: To optimize this loop, you could use a “for” loop instead, like this: The “for” loop is generally faster than the “while” loop, as it avoids the overhead of incrementing a counter. You can also improve performance by pre-allocating arrays, which means creating an array with a fixed size and then adding or removing elements as needed. This can be faster than creating an array and then growing it dynamically, as it avoids the overhead of resizing the array. Finally, you can improve performance by using the “continue” and “break” statements wisely. The “continue” statement skips the rest of the current iteration of the loop and moves on to the next iteration, while the “break” statement exits the loop entirely. By using these statements appropriately, you can minimize the number of iterations and improve performance.
8. Use Type Hints
Type hints are a way to specify the data type of a variable in GDScript. By using type hints, you can help the Godot engine optimize code and improve performance. To use type hints, simply specify the data type of a variable when you declare it, like this:
Use a type hint to specify the data type of a variablevar my_int: int = 0Type hints are optional in GDScript, but they can be useful for improving performance, as they allow the Godot engine to optimize code more effectively.
For example, let’s say you have a function that calculates the average of a list of numbers. Without type hints, the function might look like this: Type hints are optional in GDScript, but they can be useful for improving performance, as they allow the Godot engine to optimize code more effectively. For example, let’s say you have a function that calculates the average of a list of numbers. Without type hints, the function might look like this: This function works fine, but it may not be as efficient as it could be. By using type hints, you can help the Godot engine optimize the code and improve performance. Here is the same function with type hints: By specifying the data types of the “sum” and “number” variables, you can help the Godot engine optimize the code and improve performance.
9. Use the Godot Profiler
The Godot profiler is a tool that allows you to analyze the performance of your GDScript code and identify bottlenecks. To use the profiler, simply run your game or app in the Godot editor and then open the profiler window. The profiler will show you a breakdown of the time and resources used by different parts of your code, allowing you to identify areas that may be causing slowdowns. For example, the profiler might show you that a particular function is taking a long time to execute, or that a specific object is using a lot of memory. By analyzing the profiler data, you can identify the root causes of performance issues and take steps to optimize your code. To optimize your code based on the profiler data, you can try various techniques such as optimizing loops, minimizing the number of function calls, or using more efficient data structures. You can also try profiling different parts of your code to see how they compare in terms of performance.
10. Use Multithreading
Multithreading is a way to allow a program to run multiple tasks concurrently, taking advantage of multiple CPU cores. In GDScript, you can use the “Thread” class to create and manage threads. To use multithreading, create a new class that extends “Thread” and define a “_thread_func” function that contains the code you want to run in the thread. Then, create an instance of the class and call the “start” function to start the thread. Here is an example of how to use multithreading in GDScript: Multithreading can be a powerful tool for improving the performance of tasks that are CPU-intensive or that require a lot of data processing, such as loading data or performing complex calculations. By running these tasks in separate threads, you can take advantage of multiple CPU cores and improve performance.
Conclusion
In conclusion, these are the top 10 GDScript tips for improving performance in your games and apps. By following these best practices and techniques, you can optimize your code and ensure that it runs smoothly and efficiently. It’s worth noting that optimizing code can be a complex and time-consuming task, and it may not always be necessary, depending on the specific requirements of your project. However, by following these tips and using tools like the Godot profiler, you can ensure that your GDScript code is as efficient as possible and avoid performance bottlenecks. This content is accurate and true to the best of the author’s knowledge and is not meant to substitute for formal and individualized advice from a qualified professional. © 2022 Michael McGuire
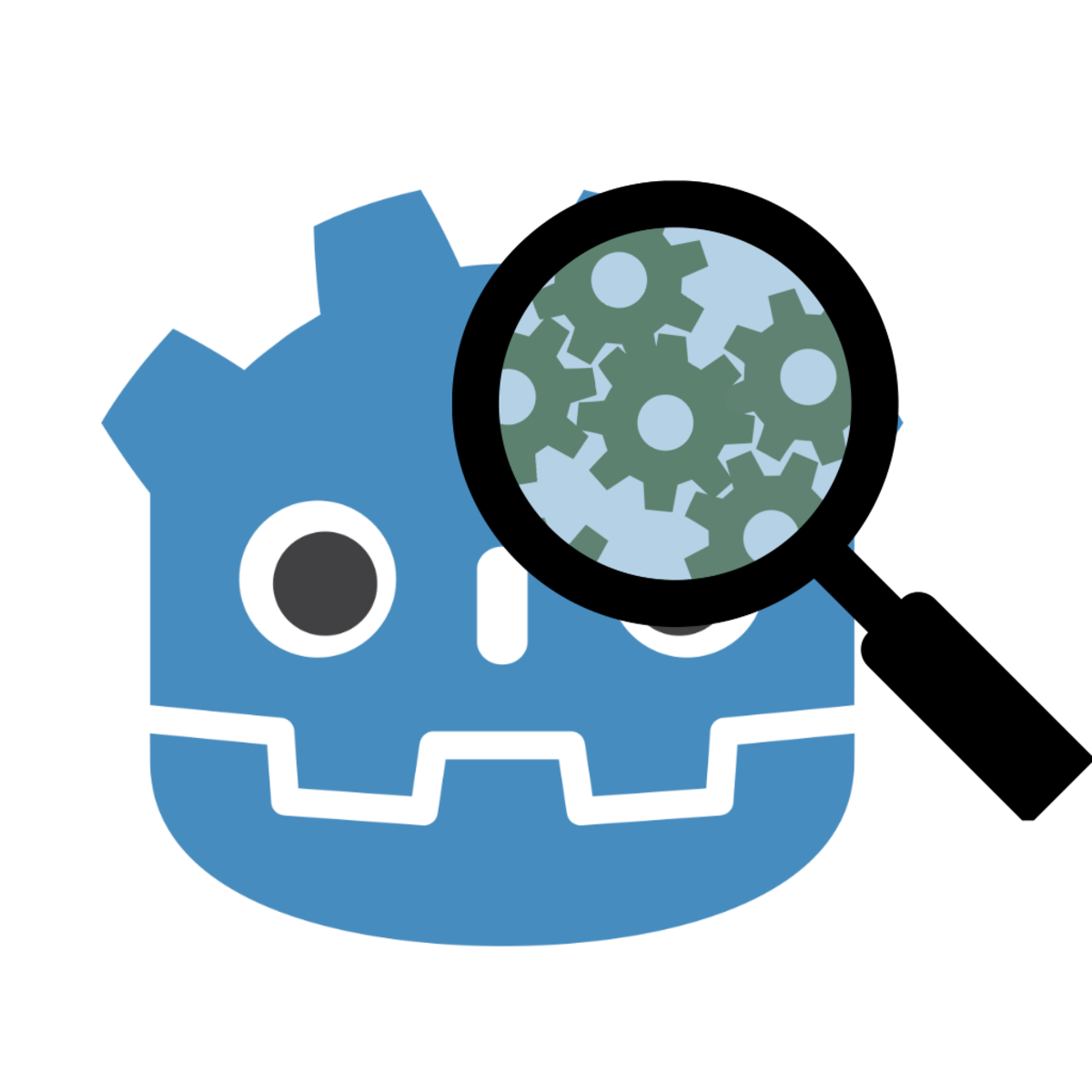